Python Tips Series: Tuples and Named Tuples
- Maia
- Oct 15, 2020
- 2 min read
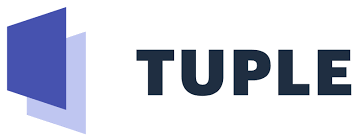
When we talk about tuples, we normally think of tuples are read-only list or immutable list. That's absolutely right. And, there's nothing wrong with saying that. However, there are more in tuple than just that.
In this series, I want to look at tuple as a data structure and how it is normally used as data objects in an application.
But first of all, let's review the basic characteristic of tuple. As we know:
- Tuples are container objects that contain other objects
- Tuples are indexable that we can access its elements by using square bracket syntax []
- Tuples are iterable that we can use it loops
- Tuples are immutable (read-only)
Let's define a basic tuple that represent a point in a 2D space and explore it.
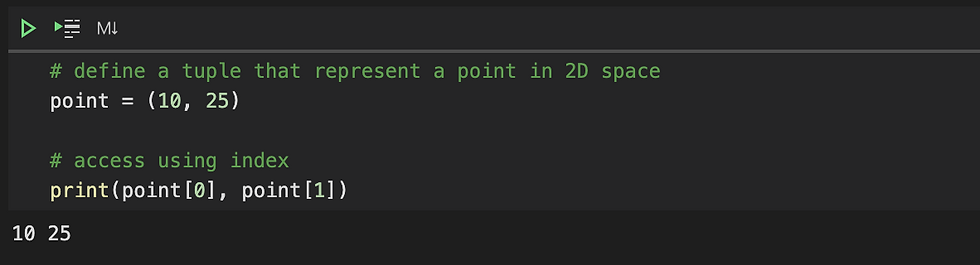
We can iterate through the tuple's elements similarly to a list.

However, normally when we use tuple, like in this case, the tuple index has a very specific meaning. In this case, first element means the x value on the x-axis and the second element is the y value on the y-axis. So, we often unpack it.
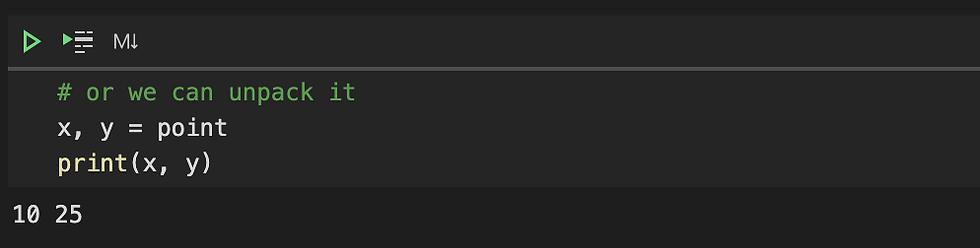
Now, let's define a little more complex tuple that represent a stock price data point.

We can unpack the tuple using the wildcard (*) operator to ignore some elements that we don't care for now. For example, if we only care about the stock symbol and the Close price, then we can do this.
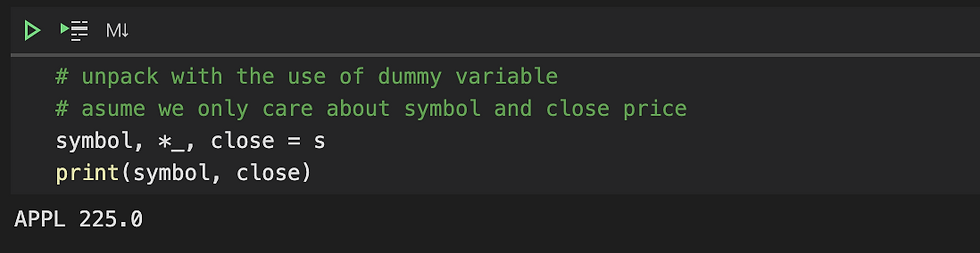
Notice that we use the dummy variable (the underscore symbol _) here, but in fact we can actually use any variable name, it just means we don't care about those values.
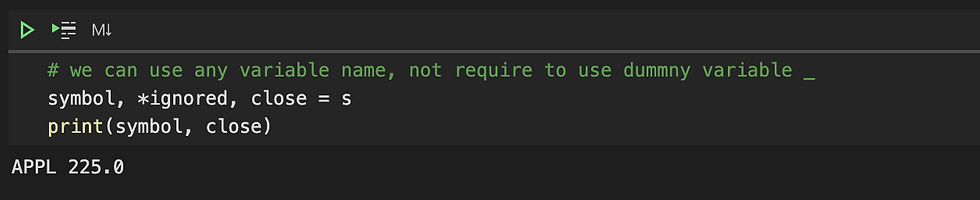
The more interesting thing about tuple is we can use tuples as named tuples and can access its elements using attributes just like class or using key/value syntax just like dictionary.

Now, we can access p1 and p2 using tuple index.
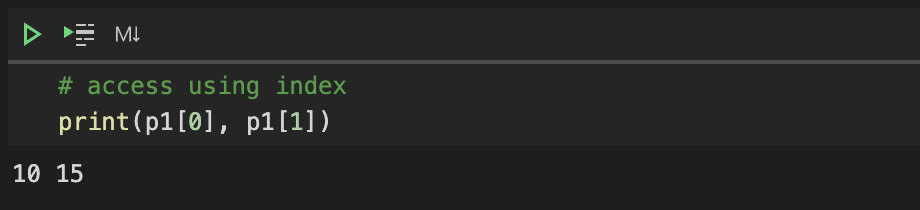
Or, we can access using dot notation of class attribute syntax.
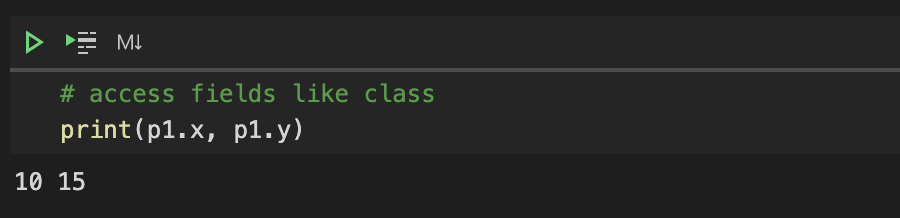
We can compare p1 and p1 by equality or identity.
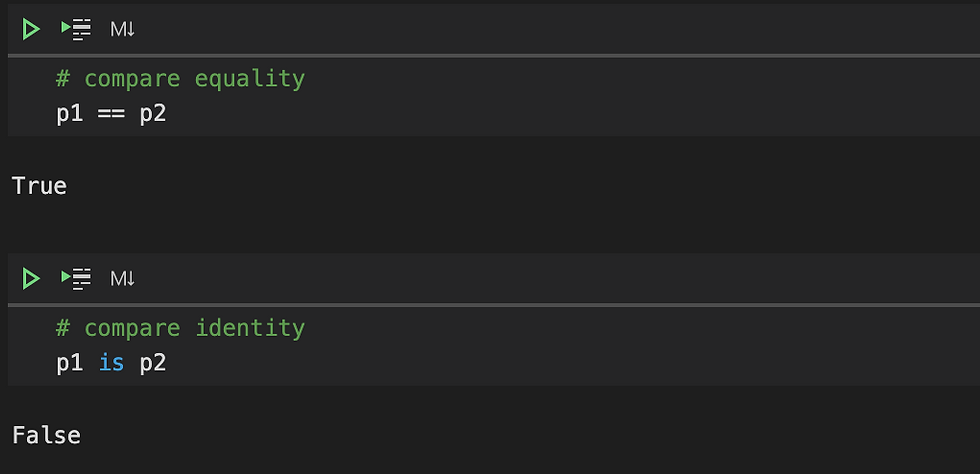
We can also convert the tuple to dict to access its element using dict key syntax.
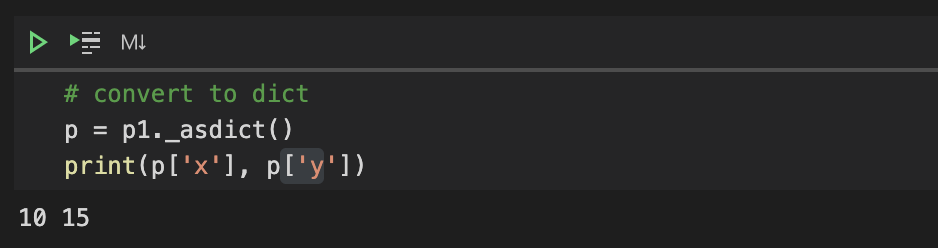
That's all about some tips I found very useful about tuple and named tuples that I want to share today.
Happy coding!
Comments