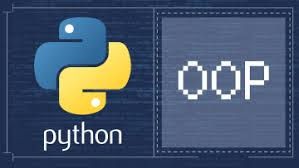
Object Oriented Programming or OOP is probably the most important programing concept that all programmers needs to know. When we begin learning Python, mostly we start with procedural programming which is writing some functions and calling functions. But in reality, most of sizable data science projects, especially all of the major open-source Python libraries are using OOP.
Therefore, to be able to work on a real project and be able to write and understand real-life Python code we all need to know and at least master the basics of OOP in Python.
Today, I will share some very key fundamental concepts of Python OOP. These will build a very strong foundation to understand object oriented programming in Python.
So, let's get started with the most basis concept, of course, what is an Object?
From a simplest point of view, an object is like a container which contains data and action.
Object's data are also called states or attributes, and object's actions are also called behaviors or methods.
For example, we if we call Dog is an object, then a Dog object can have some data like color or age, etc; and a Dog object can perform some actions like walk, bark, etc.
How do we create objects in Python? By defining a class first.
So, what is a class? A class is a blueprint or template to create objects. Any object must be belonged to a class.
After we define a class, we then can create a object from it, that process call instantiation and an object is called an instance of that class.
Enough theory, let's create the most simplest Python object in the world, called Dummy class.
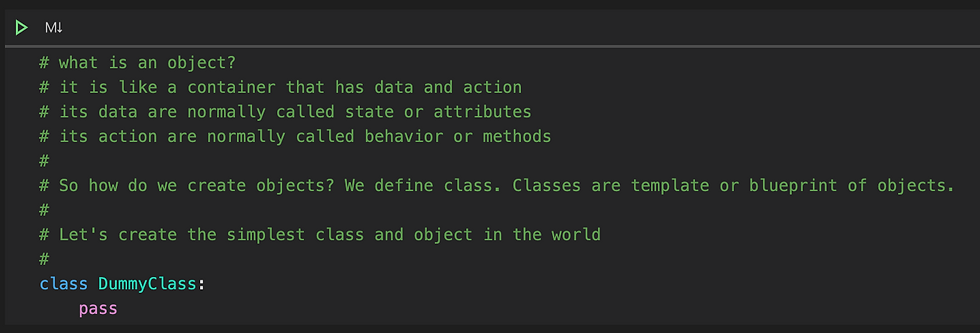
We define a class by using a keyword 'class'. This 'DummyClass' does not have anything inside it at all. We didn't give it any data or behavior at all. But it is still a valid and legitimate class in Python.
And, interestingly, it has lots of thing more than we might thought.
Let's explore that type it is.
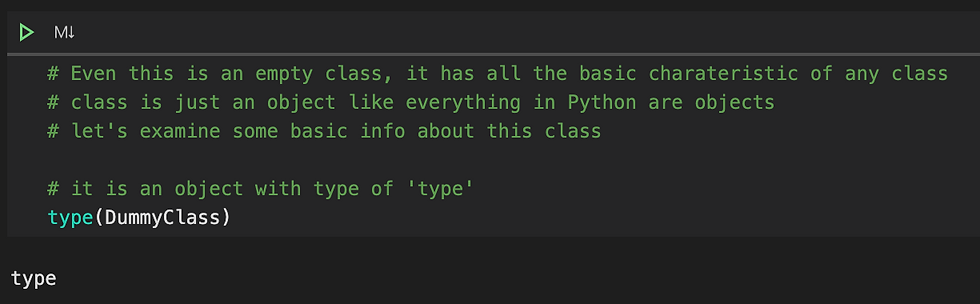
It turns out it has a type of 'type' which also means 'class'.
And, we can access to its name programmatically by accessing its attribute: __name__

Now, let's create an object from this class or instantiate a DummyClass object.
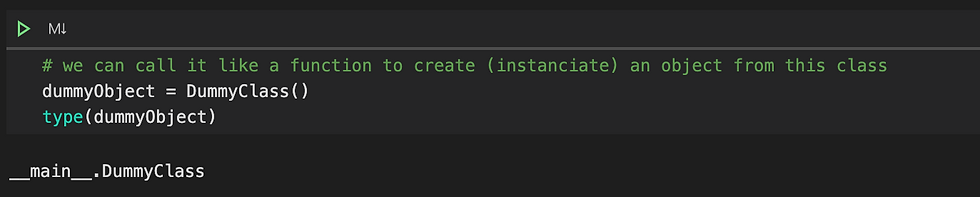
We instantiate an object by calling the class similarly like we call a function by using the parenthesis.
And, we can see that the instance of DummyClass has the type of DummyClass (of course!).
But sometimes, we are not sure if an object is an instance of a class or not, we can use function isinstance() to check.

Now, let's create a class with a little more information.
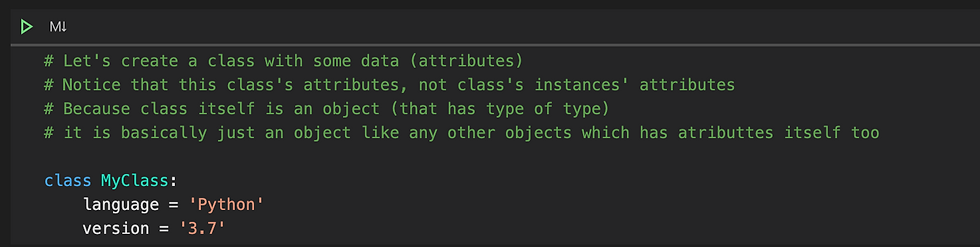
We define two data or attributes to MyClass, and notice that these are attributes of the class, not attributes of its instances.
We can get attributes of class by calling getattr() function.

And, it's simpler to just use a dot notation instead of using getattr() function.
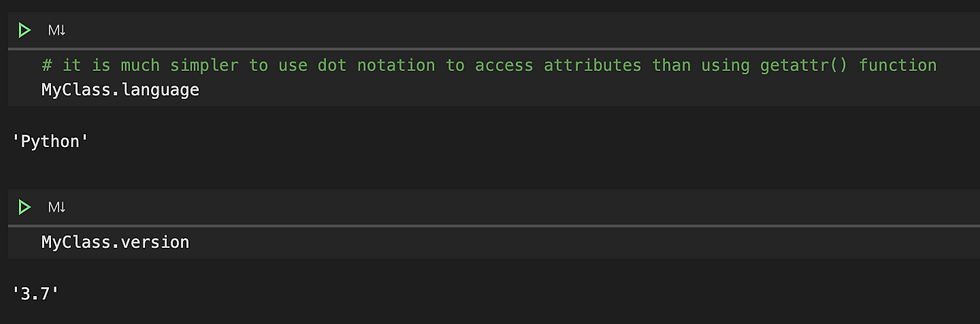
We can also dynamically set attributes to the class by using setattr() function, or use the dot notation similarly like getattr().
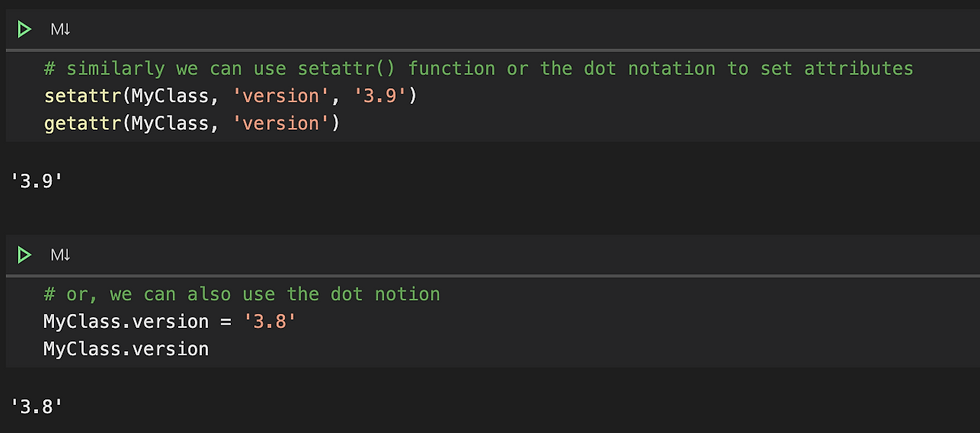
And, it's very powerful that we can add new attributes any time we want.
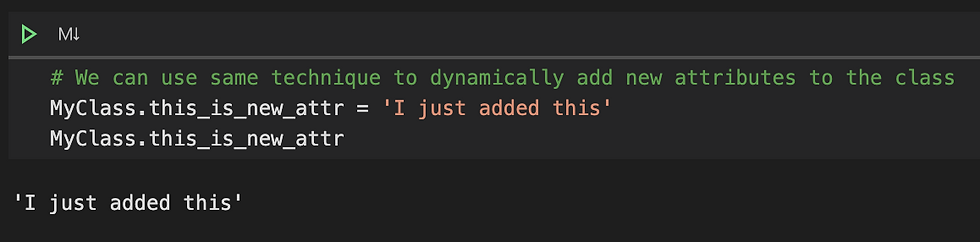
So, where do these attributes go? Actually, Python stores them in the dictionary __dict__ that we can access any time, even we rarely and should not do that.
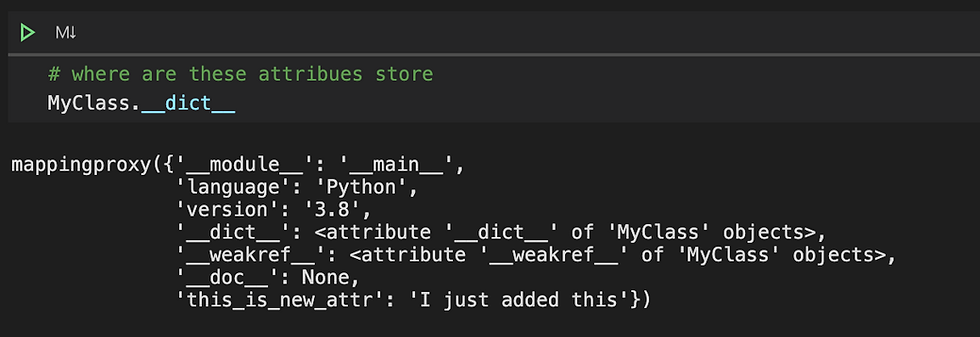
That's all for today about Class in Python OOP. Next times, we will explore deeper about the Object.
Happy OOPing!
Comments